Introduction
In this post, we are going to see how to create a dictionary in ansible during the playbook execution dynamically and how to add items or elements to a dictionary.
Before going further, let us refresh our memory of knowledge on the dictionaries. Especially python dictionaries because Ansible is pythonic and the word dictionary was introduced in python. in Perl, it used to be called as hash
Dictionaries are a collection of key: value
data sets. changeable, indexed and unordered.
the difference between a list(array) and a dictionary is in the dictionary every value has a name(key) and not indexed by their positional values like 0,1,2 etc
Hope the following picture would give a quick idea about the dictionaries.
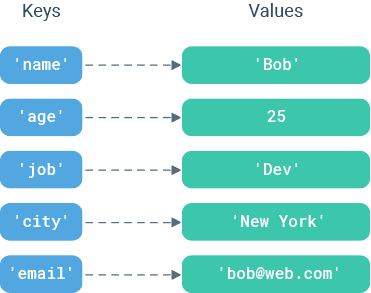
I am sure, if you are here then you must have an idea about dictionaries anyway. So let's proceed.
How to Create Dictionaries in Ansible
There are multiple ways to create a dictionary in ansible and we would be seeing all of them with examples.
Creating a Dictionary under vars
section in Ansible
You can declare and create a dictionary using the normal variable declaration methodology of Ansible. you can place your dictionary variable under the vars
segment of your playbook like this
In the following playbook, you can see that we have created a dictionary named mydict
and stored some key-value data and displaying it using the debug statement
---
- name: Dictionary playbook example
hosts: localhost
vars:
- mydict: {
'Name': 'Sarav',
'Email': 'aksarav.middlewareinventory.com',
'location': 'chennai'
}
tasks:
- name: Display the Dictionary
debug: var=mydict
execution result of this playbook would be as follows
➜ ansible-playbook Dict.yml
PLAY [Dictionary playbook example] *****************************************************************************************************************************************************************************
TASK [Gathering Facts] *****************************************************************************************************************************************************************************************
ok: [localhost]
TASK [Display the Dictionary] **********************************************************************************************************************************************************************************
ok: [localhost] => {
"mydict": {
"Email": "aksarav.middlewareinventory.com",
"Name": "Sarav",
"location": "chennai"
}
}
PLAY RECAP *****************************************************************************************************************************************************************************************************
localhost : ok=2 changed=0 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
you can create an empty dictionary as well by not adding any values inside the parenthesis during the declaration like this.
--- - name: Dictionary playbook example hosts: localhost vars: # Dictionary with some values - mydict: { 'Name': 'Sarav', 'Email': 'aksarav.middlewareinventory.com', 'location': 'chennai' } #Empty Dictionary - mydict2: {} tasks: - name: Display the Dictionary debug: var=mydict2
Creating a Dictionary in Ansible using the default
function
You can also create a dictionary within the task itself and define some variable as a dictionary by default, the default value kicks in when there was no previous declaration for the same variable.
For example. If the variable has not been declared earlier using the vars
method, you can directly set them as a dictionary by default. you can define some value to it by default using the default
This is much more helpful while creating variables in a dynamic manner. To declare the variable within the task we use set_fact
module.
--- - name: Dictionary playbook example hosts: localhost tasks: - name: Creating a Dictionary in a Task set_fact: # Dictionary with some value mydict2: "{{ mydict2 | default({ 'Name': 'Sarav'})}}" # Empty Dictionary mydict: "{{ mydict | default({})}}" - name: Display the Dictionaries debug: var="{{item}}" loop: - 'mydict2' - 'mydict'
this playbook would return the following result upon execution
➜ ansible-playbook Dict.yml
PLAY [Dictionary playbook example] ******************************************************************************************************************************************************
TASK [Gathering Facts] ******************************************************************************************************************************************************************
ok: [localhost]
TASK [Creating a Dictionary in a Task] **************************************************************************************************************************************************
ok: [localhost]
TASK [Display the Dictionaries] *********************************************************************************************************************************************************
ok: [localhost] => (item=mydict2) => {
"ansible_loop_var": "item",
"item": "mydict2",
"mydict2": {
"Name": "Sarav"
}
}
ok: [localhost] => (item=mydict) => {
"ansible_loop_var": "item",
"item": "mydict",
"mydict": {}
}
PLAY RECAP ******************************************************************************************************************************************************************************
localhost : ok=3 changed=0 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
How to Append or Add items into Ansible Dictionary
In this segment, we are going to see how to append or add items into Ansible dictionary and how to create a list of dictionaries (or) List with nested dictionaries.
We have seen how to declare dictionaries in python so far and now we are going to see how to add new items (or) a key, value dataset into an existing ansible dictionary.
One way to add/append elements is during the declaration time which we have seen in the last two examples. in this section, we are going to see how to add or append new elements into dictionaries dynamically at runtime
In the following playbook example, we are going to create a dictionary named userdata using the default filter and adding elements to it using the combine
filter
---
- name: Dictionary playbook example
hosts: localhost
tasks:
- name: Create and Add items to dictionary
set_fact:
userdata: "{{ userdata | default({}) | combine ({ item.key : item.value }) }}"
with_items:
- { 'key': 'Name' , 'value': 'SaravAK'}
- { 'key': 'Email' , 'value': '[email protected]'}
- { 'key': 'Location' , 'value': 'Coimbatore'}
- { 'key': 'Nationality' , 'value': 'Indian'}
- name: Display the Dictionary
debug: var=userdata
So we have a list of items (datasets) with key and value stored in our playbook and we are iterating one by one and referring them as item.key
and item.value
and adding one by one using the combine
filter
at runtime, the items would be appended into the userdata
dictionary and we are displaying it using the debug module. Here is the execution result of this playbook
One more best example of this dynamic dictionary creation is in our other article, where JSON data gets processed and created as a phonebook using ansible dictionary. Refer the article
Here is the playbook for your quick reference
---
- name: JsonQuery Playbook
hosts: localhost
tasks:
- name: Download JSON content play
uri:
url: https://jsonplaceholder.typicode.com/users
return_content: yes
register: jsoncontent
- name: Collecting UserName and Mobile Number info
no_log: True
set_fact:
phonebook: "{{phonebook|default({}) | combine ( {item.name : item.phone}) }}"
with_items: "{{ jsoncontent.json | json_query('[*]')}}"
- name: The Phonebook
debug: var=phonebook
How to create List of Dictionaries in Ansible
In this segment, we are going to see how to create a List of dictionaries. To put it in other words, a list with dictionaries in it.
The following diagram depicts the structure of an idle List of dictionaries. We have a list of three dictionaries stored inside an array or list.
Let us see how to create this data structure, List of dictionary with Ansible
---
- name: Dictionary playbook example
hosts: localhost
tasks:
- name: Create and Add items to dictionary
set_fact:
userdata: "{{ userdata | default([]) + [{ 'Name' : item.Name, 'Email' : item.Email, 'Location' : item.Location }] }}"
with_items:
- { 'Name': 'Sarav' , 'Email': '[email protected]', 'Location': 'Chennai'}
- { 'Name': 'Richa' , 'Email': '[email protected]', 'Location': 'Chennai'}
- { 'Name': 'Hanu' , 'Email': '[email protected]', 'Location': 'Hyderabad'}
- name: Display the Dictionary
debug: var=userdata
in this playbook, we are creating a variable named userdata
and declaring it as a list (array) and adding each dictionary (key-value datasets) we have stored inside with_items
Like the previous example, we do not need any functions like combine
to add a new element to list (or) array, this can be simply done with the +
plus sign
Working with Ansible Dictionaries - Examples
I have written various articles on how to process and iterate and filter ansible dictionaries. We have given the hyperlinks here for you to read further.
-
How to process (or) iterate through the ansible dictionary
-
A real-time example of a complex Nested List and Dictionaries of Ansible
-
A JSON data processing with Dictionary techniques and Json_query ansible
-
How to handle Data processing Errors with Ansible, Dict Object
-
How to Filter and Select elements from Dictionary using Ansible select_attr
-
Ansible Map Examples - Filter List and Dictionaries
How to work with Ansible List - Create List, Append items
So far we have talked about Ansible Dictionary. The other famous datatype is Ansible List and you might use both of them together often.
I have written an article on Ansible List too. Check it out.
Ansible List Examples – How to create and append items to List
Cheers
Sarav AK சரவணன் அ.க
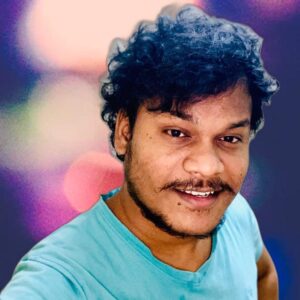
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content