Ansible template module helps to template a file out to a remote server.
Simply put, at runtime ansible template module updates the jinja2 interpolation syntax variables with actual values and copy the template file to the remote server with the specified name.
This is most useful for configuration templating and Server provisioning. We can see more Ansible template and interpolation syntax and Ansible template can be used in detail.
What does Ansible template module do
Ansible template module does two things
- Replace the Jinja2 interpolation syntax variables present in the template file with actual values
- copy (scp) the file to the remote server.
Ansible template module is designed to perform only these two things and it does the same perfectly and before going to Ansible template it is necessary that we should understand the Ansible interpolation syntax and how it works.
Ansible Interpolation Syntax and Variables
To understand the Ansible template it is necessary that you Ansible interpolation syntax and variables and how it works.
Let us consider the normal shell script where you would have to pass the value of some variable during the time of execution. For example
#!/bin/bash
# Script find and type of files which are N days older
#
if [ $# -ne 3 ]
then
echo Please Check and Rerun
echo – -----------------------
echo "$0 Directory FileExtension DaysOld"
echo $0 /var/log/tomcat "*.log" 30
exit
fi
DIR=$1
FILEEXT=$2
DAYSOLD=$3
find ${DIR} -type f -name ${FILEEXT} -mtime +${DAYSOLD}
the preceding script is to simply perform some find command it takes all its input from the user during the runtime, till we get the right values from the user we have variable names like DIR, FILEEXT, DAYSOLD
as a placeholder
when we start the script with the following command
./test.sh "/apps/tomcat" "*.log" 30
the variable names would be replaced by these actual values and the script would be executed as intended.
Now in the world of Ansible, let's suppose you want to perform the same task and get the inputs from the user at run time as variables.
Here is some simple playbook
While starting this playbook with ansible-playbook
command you have to declare the variables just like you did with Shell script. this is what is called interpolation.
In Ansible {{ }}
is the interpolation syntax whereas in shell script it is ${ }
Finally, you can start your playbook like this with the variables at runtime.
ansible-playbook findtest.yaml -e "DIR=/apps/Tomcat FILEEXT=*.log DAYSOLD=30"
Quick Example of Ansible template module
we are going to make some modifications in our previous shell script and going to the change the interpolation from Shell ${ }
into Ansible Jinja 2 interpolation syntax {{ }}
Shell Script - A Source file with Jinja2 interpolation
#!/bin/bash # Script find and type of files which are N days older find {{DIR}} -type f -name {{FILEEXT}} -mtime +{{DAYSOLD}}
test.sh
or test.sh.j2
the J2 is an identifier and file extension for Jinja2 files but it's not mandatory to save the source/template file with that extension.Ansible Playbook with Ansible template
---
- name: Template Example
hosts: hostgroup1
tasks:
- name: Template module to interpolate variables and copy the file
template:
src: test.sh
dest: /tmp/test.sh
owner: vagrant
group: vagrant
mode: 0755
- name: Execute the script
become: true
shell:
/tmp/test.sh
register: scroutput
- debug: var=scroutput.stdout_lines
You can notice that there two tasks in the playbook
- Ansible template module to take care of the interpolation and scp
- Ansible shell module to invoke the script once the file is placed there.
Execution output of this Playbook
Ansible Template file is used for various configuration files and to achieve configuration templating. The following example would give you a clear picture if you still have questions on the ansible template.
Configuration Templating with Ansible Template Example
Ansible templating is widely used in Ansible roles for those who are not aware of what is ansible roles, it would be little hard to grab but if you do it a couple of times you can easily understand I bet.
So I have taken the Apache httpd server setup as an example. We have created ansible role for installing and configuration apache httpd server it performs everything right from downloading the epel
repo and installing apache
The setup-apache
role is also designed to download the current and live copy of middlewainventory.com
and create an index.html
file for the apache virtual host
Github Repo URL : https://github.com/AKSarav/Ansible-SetupApacheRole
File and Folder structure of my local machine where am testing this role
here template-ex2.yml
is our main playbook which invokes the role and here is the content of that file
- –
- name: Apache2 Setup
hosts: hostgroup1
handlers:
- include: handlers/handlers.yaml
roles:
- setup-apache
Here is the content of our setup-apache/tasks/main.yml
content which is heart of our role does all the job for us
---
- name: install the EPEL release rpm
become: yes
become_user: root
yum:
name: http://dl.fedoraproject.org/pub/epel/epel-release-latest-7.noarch.rpm
state: present
- name: Ensure Apache is at the Latest version
become: yes
become_user: root
tags:
- web
yum:
name: httpd
state: latest
- name: "Create sites-available and sites-enabled Directories"
become: yes
become_user: root
tags:
- web
file:
dest: "{{ item }}"
owner: root
group: root
mode: 0644
state: directory
with_items:
- "/etc/httpd/sites-enabled"
- "/etc/httpd/sites-available"
- name: Modify the Default Apache Configuration file
become: yes
become_user: root
tags:
- web
template:
src: "{{ item.src }}"
dest: "{{ item.dest }}"
owner: root
group: root
mode: 0644
with_items:
- {src: 'basic_vh.conf.j2', dest: '/etc/httpd/sites-available/{{ domain }}.conf'}
- {src: 'httpd.conf.j2', dest: '/etc/httpd/conf/httpd.conf'}
- name: Create the Document Root Direcotory
become_user: root
become: yes
tags:
- web
file:
dest: "{{ docroot }}"
mode: 0755
owner: apache
group: apache
state: directory
- name: Symlink the sites-available VH file to sites-enabled
become: yes
become_user: root
tags:
- web
file:
src: "/etc/httpd/sites-available/{{ domain }}.conf"
dest: "/etc/httpd/sites-enabled/{{ domain }}.conf"
state: link
notify: restart apache
- name: Make Sure mime.types file is available
tags:
- web
file:
dest: /etc/httpd/conf/mime.types
state: file
register: mimeout
ignore_errors: True
- name: Copy the /etc/mime.types file to httpd conf
become: yes
become_user: root
tags:
- web
shell: "cp /etc/mime.types /etc/httpd/conf/mime.types"
when: mimeout is failed
- name: Download the home page of www.middlewareinventory.com to tmp
tags:
- web
become: yes
become_user: root
shell:
wget https://www.middlewareinventory.com
args:
chdir: "/tmp"
creates: "index.html"
- name : Changing file permission of downloaded index.html and copy to DocRoot
tags:
- web
become: yes
become_user: root
copy:
remote_src: yes
src: "/tmp/index.html"
dest: "{{docroot}}/index.html"
owner: apache
group: apache
mode: 0755
- name: Make Sure the Apache is Running
tags:
- web
become: yes
become_user: root
service: name=httpd state=restarted enabled=yes
We have a handler
which can be defined as a function
in the general programming, paradigm to declare once and to use it multiple times.
Video Ansible template example
Cheers
Sarav
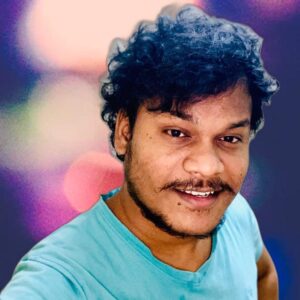
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content